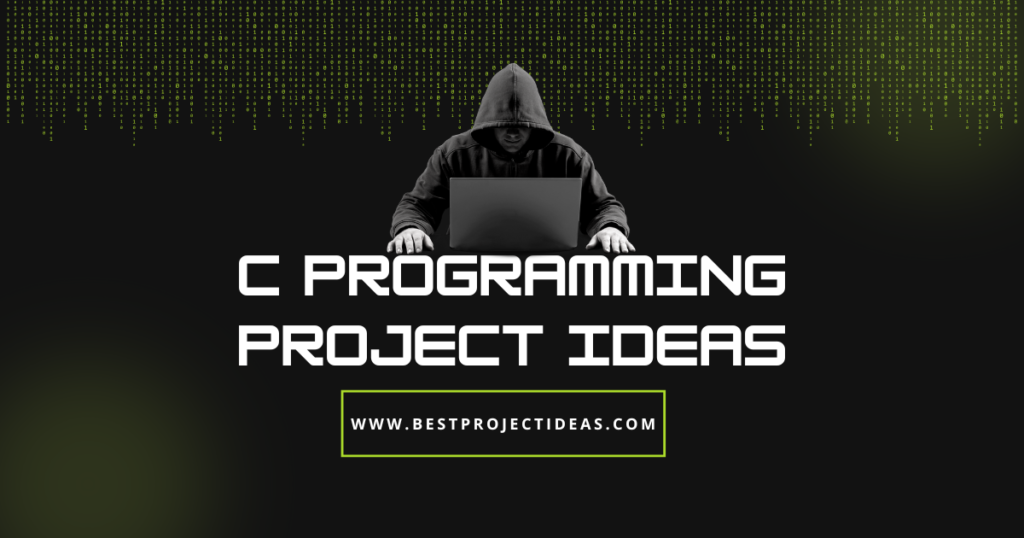
C programming is one of the most foundational languages in the world of programming.
Learning C can help you build a strong base for understanding other languages like C++, Java, or even Python. But beyond learning theory, working on C programming projects is crucial for solidifying your skills and boosting your confidence as a developer.
In this blog, we’ll dive into why C programming projects are important, the benefits of working on them, tips for choosing the right project, and a list of exciting project ideas.
Why Are C Programming Project Ideas So Important?
C programming projects help you apply the theoretical knowledge you’ve learned in a practical environment.
They challenge you to think critically and solve real-world problems. Here’s why C programming project ideas are essential:
- Hands-On Learning: They offer practical experience, which is essential for mastering any programming language.
- Problem-Solving Skills: Working on projects helps you develop problem-solving skills, as you need to figure out how to turn ideas into code.
- Career Development: Many employers look for candidates who have hands-on project experience. Having completed C projects can enhance your resume and show your potential employers that you’re serious about your programming skills.
- Understanding Core Concepts: C programming is the backbone of many advanced languages. Projects in C can help you understand core programming concepts like memory management, pointers, and data structures better.
Must Read: Amazing 499+ AI Project Ideas for Students 2024
Benefits of Doing C Programming Projects
1. Strengthens Fundamentals
Working on C programming projects helps you strengthen your understanding of core programming concepts like loops, arrays, pointers, and functions.
2. Real-World Application
You get to apply theoretical concepts in real-world scenarios, improving your ability to think logically and solve practical problems.
3. Prepares for Advanced Programming
Once you master C, it becomes easier to learn other complex languages like C++ and Java since they share many of the same principles.
4. Boosts Your Resume
Having completed projects showcases your practical experience and gives you something impressive to talk about during interviews.
5. Improves Debugging Skills
C projects can teach you how to efficiently find and fix bugs in your code, a crucial skill for any programmer.
Tips for Choosing the Best C Programming Project
- Start Simple: If you’re a beginner, start with basic projects to gain confidence. Don’t jump into complex tasks right away.
- Choose Based on Interest: Pick a project that interests you. Whether it’s gaming, automation, or file management, you’ll be more motivated if you’re excited about the topic.
- Consider Your Skill Level: Choose a project that is challenging but not impossible. Aim for a balance between learning something new and using the skills you already have.
- Look for Real-World Relevance: Select a project that solves a real problem or could be used by others. This will make it more rewarding to complete.
- Focus on Learning: While completing a project, focus on learning new concepts rather than just getting the project done.
Top 459+ C Programming Project Ideas for Students
Here’s a list of 500 C Programming Project Ideas, categorized based on difficulty levels: Beginner, Intermediate, and Advanced.
Beginner Level C Programming Projects
- Simple Calculator
- Temperature Converter
- Number Guessing Game
- Bank Account Management System
- Student Grading System
- Basic ATM System
- Simple Interest Calculator
- Area and Perimeter Calculator
- Fibonacci Sequence Generator
- Factorial Calculator
- Leap Year Checker
- Palindrome Checker
- Prime Number Finder
- Armstrong Number Checker
- Even or Odd Number Checker
- Sum of Natural Numbers
- Basic Voting System
- Array Sorter
- String Reversal
- Character Counter
- Decimal to Binary Converter
- Multiplication Table Generator
- Currency Converter
- ASCII Value Finder
- Quadratic Equation Solver
- Calculator with Switch Case
- Basic Clock Program
- Unit Conversion Program
- Vowel or Consonant Checker
- Simple Email Validator
- Reverse a Number
- Sum of Digits in a Number
- Electricity Bill Calculator
- Greatest of Three Numbers
- GCD and LCM Finder
- Time Conversion Program
- Pythagorean Triplets Finder
- Binary Search Algorithm
- Linear Search Algorithm
- Menu-Driven Program
- Armstrong Numbers within a Range
- Factorial of Large Numbers
- Merge Two Arrays
- Matrix Addition
- Matrix Subtraction
- Matrix Transposition
- Temperature Analysis Program
- Salary Calculator
- Circle Area and Circumference
- Rectangle Area Calculator
Intermediate Level C Programming Projects
- Library Management System
- To-Do List Application
- Hangman Game
- Tic-Tac-Toe Game
- Simple Student Database
- Movie Ticket Booking System
- Basic Encryption Program
- Infix to Postfix Converter
- Postfix Evaluation Program
- Simple Bank System
- Bookstore Management System
- Simple Hotel Booking System
- Address Book Program
- File Copy Program
- Linked List Implementation
- Stack Implementation
- Queue Implementation
- Tower of Hanoi Solver
- Basic Telephone Directory
- File Compression Program
- Calendar Generator
- Simple Quiz Game
- Bubble Sort Algorithm
- Insertion Sort Algorithm
- Selection Sort Algorithm
- Merge Sort Algorithm
- Quick Sort Algorithm
- Doubly Linked List
- Circular Linked List
- Polynomial Arithmetic
- Sparse Matrix Implementation
- Sudoku Solver
- Payroll Management System
- Voting System using Files
- Reverse Polish Notation Calculator
- Simple Chatbot Program
- Simple Blogging System
- Recursive Factorial Program
- Binary Search Tree Implementation
- AVL Tree Implementation
- Heap Sort Algorithm
- Radix Sort Algorithm
- Count Sort Algorithm
- Contact Management System
- Online Shopping System
- Student Report Card System
- Hospital Management System
- Maze Solver Program
- Text-Based Adventure Game
- Basic Web Server in C
Advanced Level C Programming Projects
- File Encryption and Decryption
- Basic Compiler
- Hospital Management System
- Simple Operating System Kernel
- Chess Game in C
- Memory Management System
- FTP Server in C
- Chat Application using Sockets
- File System Implementation
- Image Processing Program
- Weather Forecasting System
- Smart Traffic Light System
- Mini SQL Database Engine
- Virtual Memory Simulator
- Command Line Text Editor
- Neural Network Implementation
- Compiler for Arithmetic Expressions
- Genetic Algorithm Implementation
- Snake Game using Graphics
- Peer-to-Peer File Sharing System
- Multi-threaded Web Server
- Encryption Algorithm (AES)
- Language Translator
- Database Engine in C
- TCP/IP Client-Server Program
- File Transfer Protocol (FTP) Client
- Multi-client Chat Application
- Custom Shell Program
- HTML Parser in C
- Graph Data Structure Implementation
- Balanced Binary Tree
- Red-Black Tree
- Graph Coloring Algorithm
- N-Queens Problem Solver
- Polynomial Solver using Linked Lists
- Huffman Coding Compression Algorithm
- Page Replacement Algorithm
- Disk Scheduling Algorithm
- Matrix Chain Multiplication Solver
- Data Encryption Standard (DES)
- File Sharing with BitTorrent
- Sudoku Solver using Backtracking
- Music Player in C
- PDF Reader in C
- Traffic Simulation Program
- Packet Sniffer Program
- Mini Linux Shell
- Operating System Process Scheduler
- Online Exam System
- Airline Reservation System
Game Development Projects in C
- Ping Pong Game
- Minesweeper Game
- Snake Game
- Pac-Man Game
- Space Invaders Game
- Tetris Game
- Chess Game
- Checkers Game
- Racing Car Game
- Memory Puzzle Game
- Battleship Game
- Text-Based RPG Game
- Connect 4 Game
- Sudoku Game
- Number Puzzle Game
- Zombie Shooter Game
- Ludo Game
- Labyrinth Game
- Rock-Paper-Scissors Game
- Simon Says Game
File Handling Projects
- File Reader and Writer
- Log File Analyzer
- File Merger Program
- File Splitting Program
- File Backup System
- Text File Encryption
- Student Record System using Files
- Employee Management System
- Simple File Compression
- Journal Entry Program
- Data Log System
- Simple File Explorer
- Directory Traversal Program
- File Version Control System
- File Integrity Checker
- Personal Diary Program
- File-Based Voting System
- Encrypted File Storage System
- Simple File Search Program
- File Deduplication Program
Data Structure Projects
- Stack using Arrays
- Queue using Arrays
- Circular Queue using Arrays
- Priority Queue
- Linked List Operations
- Circular Linked List
- Doubly Linked List
- Sparse Matrix Representation
- Graph Representation
- Tree Traversal Algorithms
- Binary Search Tree Operations
- AVL Tree Implementation
- Splay Tree
- Trie Data Structure
- Red-Black Tree
- B-Tree
- Disjoint Set Implementation
- Dijkstra’s Algorithm
- Bellman-Ford Algorithm
- Kruskal’s Algorithm
Mathematical Projects in C
- Prime Number Generator
- Factorial Calculator
- Fibonacci Series Generator
- GCD and LCM Finder
- Armstrong Number Finder
- Matrix Operations (Addition, Multiplication)
- Complex Number Calculator
- Polynomial Arithmetic
- Magic Square Program
- Perfect Number Finder
- Palindrome Number Checker
- Triangle Solver
- Mean, Median, Mode Calculator
- Matrix Determinant Finder
- Vector Addition
- Binary to Decimal Converter
- Decimal to Hexadecimal Converter
- Power Function Implementation
- Modular Exponentiation
Networking Projects
- TCP Chat Server
- UDP Chat Server
- File Transfer System
- HTTP Web Server
- Telnet Client
- Simple DNS Resolver
- Email Client in C
- Ping Command Implementation
- Multi-threaded Chat Application
- Remote Command Execution
- Proxy Server
- Custom FTP Client
- Network Packet Sniffer
- Load Balancer
- Secure File Transfer
- IP Spoofing Program
- Client-Server Calculator
- Video Streaming System
- Distributed File System
- Network Monitoring Tool
Graphics-Based Projects
- Drawing Shapes Program
- Simple Animation Program
- Graph Plotting
- Fractal Generation
- Paint Application
- Line Drawing Algorithm
- 3D Cube Rotation
- Moving Car Simulation
- Bouncing Ball Simulation
- Simple Game in C using Graphics
- Maze Game
- Snake Game with Graphics
- Shooting Game
- Simple 2D Shooter
- Racing Car Game
- City Road Traffic Simulation
- Simple Image Viewer
- Flight Simulation
- Solar System Model
- Weather Animation
Algorithmic Projects
- Sorting Algorithms Comparison
- Searching Algorithms Comparison
- Shortest Path Algorithms
- Minimum Spanning Tree
- Knapsack Problem Solver
- Traveling Salesman Problem
- Longest Common Subsequence
- Matrix Chain Multiplication
- Ford-Fulkerson Algorithm
- N-Queens Problem
- Tower of Hanoi
- Backtracking Algorithm Examples
- Greedy Algorithm Examples
- Dynamic Programming Examples
- Divide and Conquer Algorithms
- Permutation Generator
- String Matching Algorithms
- Huffman Coding Algorithm
- Cryptography Algorithms
- Graph Traversal Algorithms
Database Projects in C
- Simple Database System
- Student Database Management
- Hospital Record System
- Airline Booking System
- Library Management System
- Hotel Reservation System
- Inventory Management System
- Banking Management System
- Employee Record System
- Bookstore Management
- Car Rental System
Real-World System Projects
- Payroll Management System
- Parking Management System
- Hospital Management System
- Bus Reservation System
- Railway Ticket Reservation System
- Simple E-Commerce Platform
- Stock Management System
- Hospital Billing System
- Online Food Ordering System
- Personal Finance Manager
Cryptography and Security Projects
- Caesar Cipher Program
- RSA Encryption Program
- MD5 Hash Function
- AES Encryption Algorithm
- Blowfish Encryption
- Simple Password Manager
- File Encryption System
- Digital Signature Implementation
- Data Integrity Checker
- Secure Messaging System
System Programming Projects
- Simple Command Line Shell
- Linux Process Scheduler
- Process Management System
- Memory Management System
- File System in C
- Bootloader Implementation
- Multi-threaded System
- Inter-process Communication
- File Permissions System
- Custom File System
Multithreading Projects
- Thread Pool Implementation
- Dining Philosophers Problem
- Producer-Consumer Problem
- Matrix Multiplication using Threads
- Banker’s Algorithm
- Merge Sort with Threads
- Thread-based Web Server
- Multi-threaded Chat Server
- Multi-threaded File Transfer
- Thread Synchronization Problems
AI and Machine Learning Projects
- Basic Neural Network
- Linear Regression Implementation
- Logistic Regression
- K-Nearest Neighbors Algorithm
- Decision Tree Algorithm
- Naive Bayes Classifier
- Random Forest Algorithm
- Simple Genetic Algorithm
- K-Means Clustering
- AI Tic-Tac-Toe
Operating System Projects
- CPU Scheduling Algorithms
- Disk Scheduling Algorithms
- Memory Allocation Techniques
- Paging and Segmentation
- Page Replacement Algorithms
- Virtual Memory Simulator
- Simple File System
- Basic Shell in C
- Deadlock Detection Algorithm
- Operating System Kernel
IoT Projects in C
- Home Automation System
- Smart Thermostat
- Smart Lighting System
- IoT Weather Station
- Remote-Controlled Car
- IoT-Based Security System
- Smart Door Lock
- IoT Health Monitoring System
- IoT Smart Parking System
- IoT Smart Waste Management
Hardware Interfacing Projects
- Traffic Light Control System
- Elevator Control System
- Temperature Control System
- LED Matrix Display
- Motion Detection System
- RFID Attendance System
- Home Security System
- Line Follower Robot
- Water Level Indicator
- Digital Clock
Compiler Design Projects
- Lexical Analyzer
- Syntax Analyzer
- Intermediate Code Generator
- Optimizer for Expressions
- Symbol Table Generator
- Code Generation for Arithmetic
- Simple Compiler
- Expression Evaluator
- Macro Processor
- Parser for Arithmetic Expressions
Data Compression Projects
- Run-Length Encoding
- Huffman Coding
- Arithmetic Coding
- LZW Compression Algorithm
- Burrows-Wheeler Transform
- Adaptive Huffman Coding
- Simple File Compression
- Image Compression Program
- Text Compression using Huffman
- JPEG Compression
Sorting Algorithm Projects
- Bubble Sort Implementation
- Selection Sort Implementation
- Insertion Sort Implementation
- Merge Sort Implementation
- Quick Sort Implementation
- Heap Sort Implementation
- Counting Sort Implementation
- Radix Sort Implementation
- Shell Sort Algorithm
- Tim Sort Algorithm
Game Development with Graphics
- Pong Game with Graphics
- Simple Platformer Game
- Space Shooter Game
- Racing Game with Graphics
- Brick Breaker Game
- Simple Shooting Game
- Zombie Survival Game
- Simple Physics Simulation
- 2D Maze Game
- Simple RTS Game
Embedded Systems Projects
- Traffic Light Controller
- Temperature Sensor Interface
- Simple Smart Watch
- Alarm Clock System
- Basic Digital Thermometer
- Smart Metering System
- Embedded Home Automation
- Embedded Vehicle Tracking System
- Wearable Health Monitor
- Embedded Security System
Simulations in C
- CPU Scheduling Simulator
- Disk Scheduling Simulator
- Memory Management Simulator
- Virtual CPU Simulator
- Packet Switching Simulation
- Simple AI Game Simulation
- Network Traffic Simulator
- Railway Reservation System Simulator
- Flight Booking Simulator
- Traffic Management Simulator
Cloud-Based Projects
- Cloud File Storage System
- Cloud Backup System
- Cloud-Based Text Editor
- Cloud-Based File Encryption
- Distributed Cloud Computing
- Cloud-Based Voting System
- Cloud-Based Education Platform
- Cloud-Based Chat Application
- Cloud File Synchronization System
- Cloud-Based Music Player
Miscellaneous Projects
- Morse Code Encoder
- String Matching Algorithm
- Dictionary Program
- Sudoku Solver
- Barcode Generator
- Scientific Calculator
- Railway Reservation System
- Simple Contact Manager
- Document Analyzer
- Shopping Cart Program
Networking & Socket Programming
- DNS Query Resolver
- HTTP Client in C
- Multi-threaded Server
- Secure File Transfer
- Peer-to-Peer File Sharing
- TCP Client-Server
- UDP File Transfer
- Simple IRC Client
- Email Client in C
- Simple Proxy Server
Artificial Intelligence Projects
- Tic-Tac-Toe AI
- Chess AI
- Pathfinding Algorithm
- Basic Chatbot
- AI for Sudoku Solver
- AI for Snake Game
- Neural Network Library
- Genetic Algorithm for Optimization
- K-Means Clustering
- Reinforcement Learning AI
System Security Projects
- Port Scanner
- Network Intrusion Detection System
- Secure Data Transfer System
- Virus Scanner
- Basic Firewall
- Secure File System
- Network Packet Sniffer
- Secure Login System
- Two-Factor Authentication System
- Data Integrity Verification Tool
Real-Time Systems
- Real-Time Clock
- Real-Time Traffic Simulation
- Real-Time Weather Monitoring
- Real-Time Data Processing System
- Real-Time Stock Price Monitoring
- Real-Time Chat Application
- Real-Time Video Streaming
- Real-Time IoT Dashboard
- Real-Time Multiplayer Game
- Real-Time Sensor Data Logger
This list provides a wide variety of project ideas that cover various aspects of C programming, from basic concepts to more advanced systems and algorithms.
Must Read: Interesting 299+ Django Project Ideas for Students
Conclusion
C programming projects are an excellent way to practice and improve your skills. They offer real-world applications, helping you solidify your understanding of fundamental concepts while preparing you for more complex programming tasks.
Choose a project based on your interest and skill level, and don’t be afraid to challenge yourself as you progress.
Whether you’re a beginner or an advanced programmer, there’s always a project that can help you grow. Happy coding!