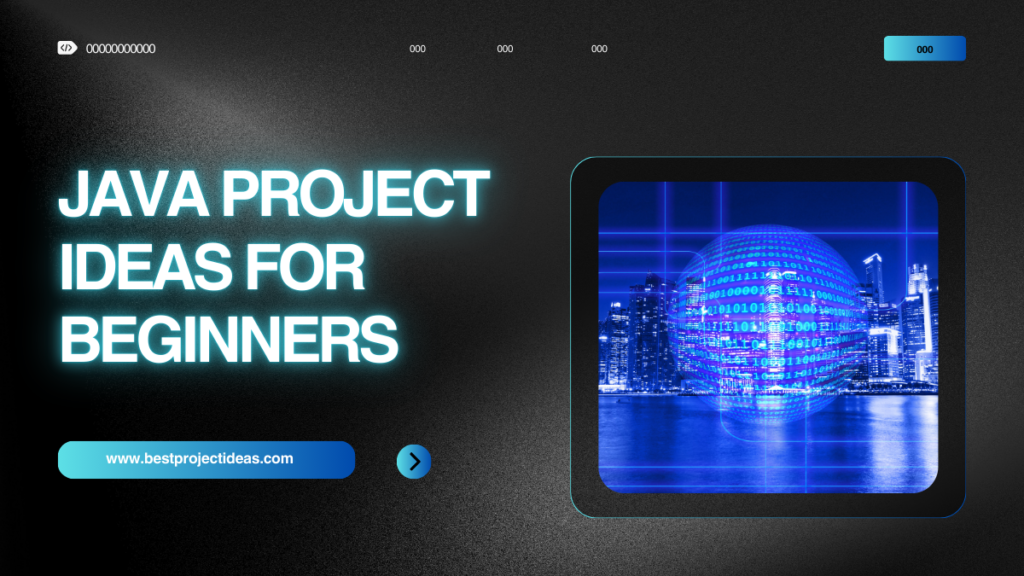
Java is one of the most popular programming languages, widely used in software development, web applications, and mobile apps. For beginners, working on Java projects is an excellent way to practice coding, understand real-world applications, and build problem-solving skills.
These projects help strengthen programming concepts, improve logical thinking, and prepare beginners for advanced development.
Must Read: Top 299+ Book Project Ideas for Students 2025-26
How to Make a Java Project as a Beginner?
If you are new to Java and want to create a project, follow these steps:
- Choose a Simple Idea – Start with basic applications like a calculator or a to-do list.
- Plan Your Project – Outline the features and functionality before coding.
- Set Up Your Environment – Install Java Development Kit (JDK) and an Integrated Development Environment (IDE) like Eclipse or IntelliJ.
- Write the Code – Implement the logic step by step.
- Test and Debug – Run your project to check for errors and fix them.
- Improve the Project – Add extra features and enhance the UI.
Benefits of Doing Java Projects
- Hands-on Learning – Projects help you apply Java concepts practically.
- Better Problem-Solving Skills – You learn how to solve real-life challenges.
- Portfolio Building – Helps in showcasing your work to potential employers.
- Improves Logical Thinking – Enhances your ability to think algorithmically.
- Prepares for Interviews – Many companies ask for project experience.
Tips for Choosing the Best Java Project
- Pick a project that aligns with your interest.
- Start with small and simple projects before moving to advanced ones.
- Ensure the project covers essential Java concepts like loops, OOP, and collections.
- Look for projects that have real-world applications.
- Choose a project that challenges you but is not too complex.
Amazing 299+ Java Project Ideas for Beginners 2025-26
Console Applications
- Basic Calculator: A command‐line calculator that performs addition, subtraction, multiplication, and division.
- Currency Converter: A console app to convert amounts between different currencies using fixed rates or an API.
- Temperature Converter: A program that converts temperatures between Celsius, Fahrenheit, and Kelvin.
- BMI Calculator: Calculate body mass index based on user input for weight and height.
- Number Guessing Game: A game where the computer randomly selects a number and the player guesses it.
- Factorial Calculator: Compute the factorial of a number using iterative or recursive methods.
- Prime Number Checker: Check whether a given number is prime or composite.
- Fibonacci Series Generator: Generate and display the Fibonacci sequence up to a specified term.
- Reverse String Program: Reverse any string input by the user.
- Palindrome Checker: Determine if a given word or phrase reads the same backward.
- Simple To-Do List: Manage a list of tasks from the console with options to add and remove items.
- Unit Converter: Convert between different units such as length, weight, and volume.
- Basic Banking System: Simulate banking operations like deposits, withdrawals, and balance checks.
- Loan EMI Calculator: Compute Equated Monthly Installments (EMIs) based on loan amount, interest rate, and tenure.
- Simple Stopwatch: Implement a stopwatch that starts, stops, and resets based on user commands.
- Countdown Timer: Create a timer that counts down from a user-specified number of seconds.
- Basic Quiz Application: A text-based quiz that asks multiple-choice questions and scores the user.
- Rock, Paper, Scissors Game: Play a classic rock-paper-scissors game against the computer.
- Simple Notepad: A command-line notepad to create, view, and edit notes.
- Calculator with History: Enhance the basic calculator by saving and displaying past calculations.
- Day of Week Finder: Determine the day of the week for any given date.
- Simple Calendar: Display the calendar for a specified month and year.
- Multiplication Table Generator: Generate and print a multiplication table for a given number.
- Vowel and Consonant Counter: Count the number of vowels and consonants in a provided string.
- Word Count Program: Count and display the number of words in a sentence or paragraph.
- Basic Alarm Clock: Set an alarm by entering a time, then notify the user when the time is reached.
- Currency Denomination Counter: Break down a given amount into currency notes and coins.
- Loan Interest Calculator: Calculate the total interest payable on a loan.
- Simple Grade Calculator: Compute grades or GPA based on input scores.
- Age Calculator: Determine a person’s age from their date of birth.
- Prime Number Generator: Generate all prime numbers up to a user-defined limit.
- Sum of Digits Calculator: Calculate the sum of all digits in a given number.
- Number Reversal Program: Reverse the digits of a number provided by the user.
- ASCII Value Finder: Display the ASCII values for characters entered by the user.
- Character Frequency Counter: Count how many times each character appears in a string.
- Binary to Decimal Converter: Convert a binary number to its decimal equivalent.
- Decimal to Binary Converter: Convert a decimal number into binary form.
- Hexadecimal Converter: Convert between hexadecimal and decimal values.
- Leap Year Checker: Determine whether a given year is a leap year.
- Simple Encryption/Decryption: Implement a basic cipher to encrypt and decrypt text.
- Matrix Addition/Subtraction: Perform addition and subtraction on two matrices.
- Array Sorting Program: Sort an array using a basic sorting algorithm like bubble sort.
- GCD Calculator: Find the greatest common divisor (GCD) of two numbers.
- LCM Calculator: Compute the least common multiple (LCM) for a set of numbers.
- Simple Interest Calculator: Calculate simple interest for a given principal, rate, and time.
- Compound Interest Calculator: Compute compound interest over time.
- Time Converter: Convert seconds into hours, minutes, and seconds.
- Temperature Trend Analyzer: Process a list of temperature readings and analyze trends.
- Basic Text Editor: Create a rudimentary console-based text editor for simple editing tasks.
- Binary Search Implementation: Implement the binary search algorithm on a sorted array.
File Handling and Data Processing
- File Reader and Writer: Read from and write to text files using Java’s I/O classes.
- CSV File Processor: Load and process CSV files to display or manipulate data.
- Log File Analyzer: Parse server log files to identify errors or trends.
- Text File Merger: Combine multiple text files into one consolidated file.
- Word Frequency Counter in File: Count how often each word appears in a file.
- File Encryption/Decryption: Encrypt a text file and later decrypt it using a simple cipher.
- File Compression Tool: Implement a basic algorithm to compress and decompress files.
- File Search Utility: Search for a particular keyword within files in a directory.
- File Backup Utility: Automatically create backup copies of specified files.
- File Renaming Utility: Batch rename files in a directory based on certain criteria.
- XML File Parser: Read and parse XML files to extract useful data.
- JSON Data Processor: Process JSON files and convert them into Java objects.
- Log File Monitor: Continuously monitor log files for changes and output alerts.
- File Splitter: Split a large file into several smaller files.
- File Merger for Large Files: Merge multiple smaller files into one large file.
- File Permission Checker: Check and display file permissions in a given directory.
- Directory Traversal Utility: Recursively list all files and subdirectories in a folder.
- File Duplicate Finder: Identify and list duplicate files within a directory.
- File Metadata Extractor: Extract and display metadata (like creation date and size) from files.
- File Integrity Checker: Calculate and compare checksums to verify file integrity.
- File Copier with Progress Bar: Copy files while showing a progress indicator.
- Batch File Processor: Apply the same processing task to multiple files in one go.
- Text File Search and Replace: Search for a term in a file and replace it with another.
- Log File Formatter: Reformat log file entries for improved readability.
- File Converter (TXT to PDF): Convert plain text files into PDF documents.
- File Timestamp Updater: Update or modify the timestamps of files.
- File System Simulator: Simulate basic file system operations such as create, delete, and move.
- CSV to JSON Converter: Convert CSV data into JSON format.
- JSON to XML Converter: Transform JSON files into XML format.
- File Archiver: Create an archive (zip) file from a group of files.
- File Permission Changer: Modify the permission settings of files through a utility.
- Large File Reader: Implement efficient methods to read very large files.
- File Size Analyzer: Calculate and report the sizes of files in a directory.
- Duplicate Line Remover: Remove duplicate lines from a text file.
- Word Search Puzzle Generator: Generate a word search puzzle based on text input.
- Log File Trend Analyzer: Analyze a log file to detect trends over time.
- File Content Reverser: Reverse the contents (line-by-line or whole text) of a file.
- File Split and Merge Utility: Provide both splitting and merging functionalities in one tool.
- File Encryption with Password: Encrypt files using a password-based encryption method.
- CSV File Sorter: Sort the rows of a CSV file based on a selected column.
- File Statistics Generator: Generate statistics (e.g., word count, line count) for a file.
- File Format Validator: Check if a file meets specific format requirements.
- File Compression Ratio Calculator: Calculate the ratio of original file size to compressed file size.
- File Path Normalizer: Convert file paths to a standardized format.
- Config File Reader: Parse configuration files (e.g., .properties) to extract settings.
- Text Analyzer: Perform basic sentiment or frequency analysis on text from a file.
- File Version Control Simulator: Simulate simple version control operations on files.
- File Change Logger: Track and log changes made to a file over time.
- Directory Size Calculator: Calculate the total size of all files within a directory.
- File System Event Notifier: Notify the user when changes occur in a monitored directory.
Data Structures and Algorithms Projects
- Array Manipulation Tool: Create utilities for common array operations like insertion, deletion, and searching.
- Linked List Implementation: Build a simple singly linked list with basic operations.
- Stack Implementation: Implement a stack using arrays or linked lists and include push/pop methods.
- Queue Implementation: Build a queue data structure and test its FIFO behavior.
- Binary Tree Traversal: Implement in-order, pre-order, and post-order traversal methods for a binary tree.
- Sorting Algorithm Visualizer: Create a tool to visualize how different sorting algorithms work.
- Searching Algorithm Visualizer: Demonstrate linear and binary search algorithms step by step.
- Recursion Practice: Solve problems using recursion, such as computing the nth term in a series.
- Graph Representation: Represent a graph using an adjacency matrix or list.
- Graph Traversal (BFS/DFS): Implement breadth-first and depth-first search algorithms on a graph.
- Sorting and Searching Library: Bundle several basic algorithms into one utility library.
- Merge Sort Implementation: Code the merge sort algorithm and test its performance.
- Quick Sort Implementation: Implement quick sort with pivot selection and partitioning.
- Heap Sort Implementation: Develop the heap sort algorithm using a binary heap.
- Insertion Sort Implementation: Code the insertion sort algorithm to sort arrays.
- Selection Sort Implementation: Write a program to perform selection sort on a list.
- Bubble Sort Implementation: Implement bubble sort and compare its efficiency.
- Hash Table Implementation: Create a simple hash table with basic put/get functionality.
- Binary Search Implementation: Code a binary search algorithm to find elements in a sorted array.
- Linked List Sorting: Implement a sorting algorithm to order elements in a linked list.
- Stack Calculator: Use a stack to evaluate postfix expressions.
- Expression Tree: Build and evaluate an expression tree from an arithmetic expression.
- Dijkstra’s Algorithm: Implement the shortest path algorithm for weighted graphs.
- Kruskal’s Algorithm: Create a program that finds a minimum spanning tree using Kruskal’s method.
- Prim’s Algorithm: Implement Prim’s algorithm for generating a minimum spanning tree.
- Topological Sorting: Order the nodes of a directed acyclic graph using topological sort.
- Sudoku Solver: Develop a backtracking algorithm to solve Sudoku puzzles.
- N-Queens Problem: Solve the N-Queens puzzle using recursion and backtracking.
- Maze Solver: Implement an algorithm to navigate through a maze.
- Word Ladder Solver: Transform one word to another by changing one letter at a time.
- Binary Search Tree Operations: Implement insertion, deletion, and searching in a BST.
- AVL Tree Implementation: Code a self-balancing AVL tree.
- Red-Black Tree Implementation: Create a simple red-black tree to learn balancing techniques.
- Graph Shortest Path Visualizer: Visually demonstrate the shortest path in a graph.
- Recurrence Relation Solver: Solve mathematical recurrence relations programmatically.
- Matrix Multiplication: Multiply two matrices and display the result.
- Spiral Matrix Generator: Generate a matrix with elements filled in spiral order.
- Pascal’s Triangle Generator: Print Pascal’s Triangle up to a given number of rows.
- Subset Sum Problem: Solve the subset sum problem using recursion or dynamic programming.
- Knapsack Problem: Implement a basic solution for the knapsack optimization problem.
- Traveling Salesman Problem (TSP): Build a brute-force solution for TSP.
- Permutations Generator: Generate all permutations of a set of numbers.
- Combinations Generator: Create a program that generates all combinations from a list.
- Anagram Finder: Find and group anagrams from a list of words.
- Frequency Counter: Count the frequency of each element in an array.
- Merge Two Sorted Arrays: Merge two sorted arrays into one sorted array.
- Binary Tree Depth Calculator: Calculate the height of a binary tree.
- Balanced Parentheses Checker: Verify if an expression has balanced parentheses.
- Factorial using Recursion: Calculate factorial values recursively.
- Power Function Implementation: Compute exponentiation recursively without using built-in functions.
GUI Applications
- Simple Notepad: Build a text editor using Java Swing with basic file operations.
- Calculator with GUI: Create a graphical calculator with buttons and a display.
- Digital Clock: Develop a digital clock that displays the current time.
- Temperature Converter GUI: Convert temperatures using a graphical interface.
- Currency Converter GUI: Build a GUI app to convert currencies with input fields and buttons.
- Unit Converter GUI: Create a graphical tool for converting various units.
- To-Do List App: Design a task manager that lets users add, update, and delete tasks.
- Tic Tac Toe with GUI: Implement a two-player tic tac toe game using Swing.
- Hangman Game GUI: Create a graphical version of the hangman word guessing game.
- Contact Manager: Develop a GUI application to add, edit, and delete contact information.
- Student Information System: Build a basic CRUD system to manage student records.
- Employee Management System: Create a desktop application to manage employee details.
- Inventory Management System: Develop a system for tracking product inventory.
- Library Management System: Build a simple app to manage library books and borrowers.
- Music Player Interface: Design a GUI for a music player that can load and play audio files.
- Photo Viewer: Create a basic image viewer that can open and display photos.
- Email Client: Develop a simple email client interface for sending and receiving messages.
- Chat Application GUI: Build a graphical interface for a chat client.
- File Explorer: Create a simple file explorer to navigate directories and view files.
- Calendar Application: Develop a calendar that displays dates and allows event scheduling.
- Drawing Application: Build a simple paint program that lets users draw shapes with a mouse.
- Puzzle Game: Create a sliding puzzle or jigsaw puzzle game with a graphical interface.
- Alarm Clock with GUI: Develop an alarm clock application with an interactive interface.
- Countdown Timer with GUI: Create a timer with a countdown feature and visual progress.
- Weather App GUI: Design a weather app interface that displays current conditions (using hardcoded or API data).
- BMI Calculator with GUI: Build a graphical BMI calculator that takes input and shows results.
- Quiz Application GUI: Create an interactive quiz app with multiple-choice questions.
- Recipe Manager: Develop an application to store and display cooking recipes.
- Expense Tracker: Build a GUI tool to log and track daily expenses.
- Personal Diary: Create a digital diary where users can add and manage daily entries.
- Password Manager: Develop a simple password storage and retrieval application.
- Flashcard Study App: Build an application to create, view, and test flashcards.
- Note Organizer: Design a GUI-based note organizer with categorization features.
- File Renamer GUI: Create a tool that allows batch renaming of files through a graphical interface.
- Color Picker Tool: Build an application to select colors and display their values.
- Drawing Pad: Develop an interactive drawing pad where users can sketch using various brushes.
- Image Resizer: Create a GUI tool to load and resize images.
- Calendar Reminder: Build an application that allows users to set reminders on a calendar.
- Budget Planner: Develop a monthly budget planner with input fields and charts.
- Loan Calculator GUI: Create a graphical tool to calculate loan repayments.
- Address Book: Build an application to store and manage mailing addresses.
- Task Scheduler: Develop a scheduler that helps users plan daily or weekly tasks.
- Chatbot Interface: Create a simple GUI-based chatbot that interacts with the user.
- News Reader App: Build an application that displays news headlines (using sample data or a news API).
- File Organizer: Design a tool that categorizes files into folders based on their type.
- Password Generator: Create a GUI tool to generate strong random passwords.
- Barcode Generator: Build an application that generates barcodes from text input.
- QR Code Generator: Develop a tool to create QR codes from URLs or text.
- Digital Whiteboard: Create an interactive whiteboard for drawing and brainstorming.
- E-book Reader: Build a basic e-book reader interface that displays text files.
Games
- Tic Tac Toe Game: Develop a classic tic tac toe game that can be played on either console or GUI.
- Hangman Game: Create a game where players guess letters to reveal a hidden word.
- Snake Game: Recreate the classic snake game with increasing difficulty.
- Minesweeper Game: Build a version of Minesweeper with a grid of hidden mines.
- Sudoku Game: Develop a sudoku puzzle game that checks for valid moves.
- Pong Game: Create a basic two-dimensional Pong game for two players.
- Brick Breaker Game: Build a game where a ball breaks bricks arranged at the top of the screen.
- Memory Matching Game: Develop a card matching game where players find pairs.
- Chess Game (Basic): Create a simplified version of chess with basic piece movement.
- Checkers Game: Implement the classic board game checkers.
- Connect Four: Build a two-player Connect Four game with a grid interface.
- Simon Says Game: Create a memory game where players must repeat a sequence of lights or sounds.
- Reaction Time Tester: Develop a game that measures how quickly a player responds to a prompt.
- Space Invaders Clone: Build a simple shooter game inspired by Space Invaders.
- Platformer Game: Create a basic 2D platformer where the player navigates obstacles.
- Runner Game: Develop an endless runner game with obstacles and power-ups.
- Racing Game (2D): Build a simple top-down racing game with user-controlled vehicles.
- Word Scramble Game: Create a game where players unscramble letters to form words.
- Quiz Game: Develop an interactive quiz game with scoring and timers.
- Card Matching Game: Create a game that involves matching pairs of cards.
- Solitaire Game: Build a simplified version of the solitaire card game.
- Dice Rolling Game: Simulate dice rolls and allow users to play a simple game of chance.
- Rock Paper Scissors Game: Develop a classic rock, paper, scissors game.
- Virtual Pet Game: Create a game where players care for a virtual pet.
- Fishing Game: Build a simple fishing simulation game with basic mechanics.
- Balloon Pop Game: Develop a game where players pop balloons before time runs out.
- Whack-a-Mole Game: Create a fun game where moles appear and the player must click them quickly.
- Doodle Jump Clone: Build a simple jumping game inspired by Doodle Jump.
- Flappy Bird Clone: Develop a version of Flappy Bird with simple controls.
- Maze Runner: Create a game that challenges players to navigate through a maze.
- Tower Defense Game: Build a basic tower defense game with simple enemy waves.
- Bouncing Ball Game: Develop a game where a ball bounces and the player controls its path.
- Memory Test Game: Create a game that challenges the user’s short-term memory with sequences.
- Puzzle Slider Game: Build a sliding puzzle game that reorders scrambled tiles.
- Word Search Game: Develop a game that presents a grid of letters to find hidden words.
- 2048 Game: Implement a clone of the popular 2048 sliding number puzzle.
- Simple RPG Battle: Create a text-based role-playing game with turn-based battles.
- Adventure Game: Develop a text-driven adventure where players choose their path.
- Trivia Game: Build a trivia game that asks random questions and keeps score.
- Platform Jumper: Create a game where players jump across moving platforms.
- Car Avoidance Game: Develop a game where the player dodges oncoming obstacles on a road.
- Block Breaker Game: Build a game similar to brick breaker with varying levels.
- Color Matching Game: Create a timed game where players match colors or shapes.
- Space Shooter: Develop a game in which players control a spaceship and shoot targets.
- Air Hockey Game: Build a simple air hockey simulation for two players.
- Word Puzzle Game: Create a puzzle game that challenges users to rearrange letters into words.
- Clicker Game: Develop an incremental “clicker” game where players earn points by clicking.
- Reaction Speed Game: Create a game that tests and improves the user’s reaction time.
- Dodge Ball Game: Build a game where the player avoids balls thrown at them.
- Maze Escape Game: Develop a time-based maze navigation game with increasing difficulty.
Networking, Web, Database, and Other Projects
- Chat Application: Create a simple client-server chat system that supports multiple users.
- Simple HTTP Server: Develop a basic web server that can handle simple HTTP requests.
- File Transfer Application: Build an application to transfer files between computers over a network.
- Email Sending Application: Create a program that sends emails using JavaMail API.
- Web Scraper: Develop a tool that extracts specific data from web pages.
- RESTful API Client: Build a client that consumes data from REST APIs.
- Social Media Feed Reader: Create an application that displays social media feeds (using sample data or APIs).
- Blog Management System: Develop a basic CMS for creating, editing, and deleting blog posts.
- Online Voting System: Build a simple voting system for online polls.
- Weather Data Fetcher: Create an application that retrieves weather data from an external API.
- Stock Market Tracker: Develop a tool to track stock prices and display trends.
- Task Management Web App: Build a web-based task manager with CRUD functionality.
- URL Shortener: Create a service that converts long URLs into shortened versions.
- Inventory Management System with Database: Develop an application to track inventory using database integration.
- Library Management System with Database: Build a system to manage library book records with a backend database.
- Student Management System with Database: Create a program to manage student data and records.
- Appointment Booking System: Develop an application to schedule and manage appointments.
- Online Examination System: Build a system for administering tests online with automated grading.
- Hospital Management System: Create an application to manage hospital operations and patient records.
- E-commerce Backend: Develop a basic backend system for an online store.
- Restaurant Ordering System: Build an application to take and manage restaurant orders.
- Online Forum: Create a simple discussion forum where users can post and reply.
- Blogging Platform: Develop a platform for users to create and share blog posts.
- Customer Relationship Management (CRM): Build a simple CRM to manage customer interactions and data.
- Project Management Tool: Create a tool to track projects, tasks, and deadlines.
- Real Estate Listing App: Develop an application to list and search for properties.
- Event Management System: Build a system to manage events, registrations, and scheduling.
- Forum Chat Integration: Enhance a forum by adding real-time chat features.
- Social Networking App: Create a basic social media application with user profiles and feeds.
- Payment Gateway Simulator: Develop a simulator that mimics payment processing for testing purposes.
- Feedback Collection System: Build an app to collect and analyze user feedback.
- File Upload/Download Manager: Create a web application to manage file uploads and downloads.
- URL Content Analyzer: Develop a tool that analyzes and summarizes the content of a given URL.
- Web-based Calculator: Build a calculator accessible via a web interface.
- Online Polling System: Create a system to conduct and manage online polls.
- Database Backup Utility: Develop a tool to backup and restore database records.
- Content Management System (CMS): Build a basic CMS for managing website content.
- Blogging Analytics Dashboard: Create a dashboard that displays analytics for blog posts.
- Job Portal: Develop an application that lists job openings and accepts applications.
- Real-time Chat Room: Build a web-based chat room supporting multiple simultaneous users.
- Data Visualization Tool: Create an app that fetches data from a database and displays charts.
- Appointment Reminder System: Build a system that sends reminders for upcoming appointments.
- Survey Management System: Develop an application for creating and managing surveys.
- E-learning Platform: Create a basic online learning system with courses and quizzes.
- Volunteer Management System: Build an application to register and manage volunteers.
- Fitness Tracker: Develop a tool to log and track fitness activities and goals.
- Recipe Sharing Platform: Create a web application where users can share and review recipes.
- Music Streaming App (Backend): Build a backend system for managing playlists and streaming music.
- Travel Planner: Develop an application that helps users plan trips with itineraries and booking details.
- Restaurant Reservation System: Create a system to manage table bookings and reservations for restaurants.
Must Read: Innovative 299+ Design Project Ideas for Students 2025-26
Additional Tips for Java Beginners
- Practice Regularly – The more you code, the better you become.
- Read Java Documentation – It helps understand different Java libraries and functions.
- Join Java Communities – Engage in online forums and discussions.
- Keep Improving – Refactor your code and add new features.
Conclusion
Java projects are a great way to enhance your skills and build confidence in coding.
By starting with small and manageable projects, beginners can gradually progress to more complex applications. Choose a project that interests you and start coding today!