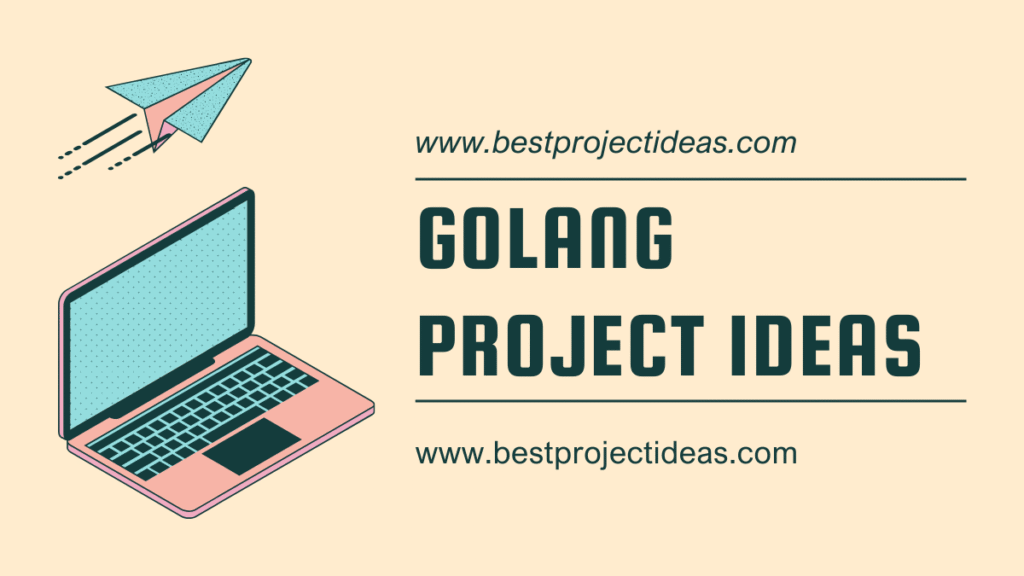
Go, also called Golang, is a modern, open‑source programming language created by Google. It’s loved for its simplicity, speed, and powerful support for handling many tasks at once. But learning Go’s syntax and features only gets you so far: real progress comes when you build something real. That’s where project ideas come in.
In this blog, you’ll discover why Go project ideas are so important, how to generate your own, and the key benefits of working on Go projects. We’ll also share practical tips for picking the best idea, essential tools and libraries to learn, and a curated list of projects—ranging from beginner to advanced levels—to kickstart your Go journey. Let’s dive in and start coding!
Must Read: Top 299+ Coding Project Ideas for Beginners 2025-26
What Is Go (Golang)?
Go, often called Golang, is a modern programming language created by Google. It’s known for being fast, simple, and great at handling many tasks at once (concurrency).
Whether you want to build web servers, command‑line tools, or network utilities, Go makes it easy and fun!
Why Are Golang Project Ideas So Important?
- Skill Growth
- Tackling real projects helps you learn Go’s unique features (like goroutines and channels).
- Portfolio Building
- A solid list of completed Go projects shows employers you can solve real problems.
- Problem‑Solving Practice
- Turning ideas into code refines your design and debugging skills.
- Community Contribution
- Good Go projects often become open‑source tools that help others.
299+ Exciting Golang Project Ideas 2025-26
Web Development
- Build a basic HTTP server using Go’s
net/http
package to serve static files. - Create a RESTful API for a TODO app using the Gin framework with full CRUD operations.
- Implement user authentication in a web app using JWT tokens and middleware in Echo.
- Develop a URL shortener microservice with Gin, storing mappings in Redis.
- Build a real‑time chat application using WebSockets and Gorilla WebSocket.
- Create a blog platform with template rendering using
html/template
and PostgreSQL. - Build an e‑commerce backend with Echo, implementing product, cart, and order endpoints.
- Develop a file upload and management service using Gin and AWS S3 integration.
- Implement server‑side rendering with Fiber and embed dynamic data.
- Build a GraphQL API using
gqlgen
, supporting queries and mutations. - Create a multi‑language website with i18n support using
go-i18n
. - Develop a CMS backend with Buffalo, including media, pages, and user roles.
- Build a forum application with authentication and threaded comments using Beego.
- Implement OAuth2 login with Google in a Gin‑based web app.
- Create a single‑page app backend with CORS handling and REST APIs.
- Build an admin dashboard that uses WebSockets for live metrics updates.
- Develop an event ticketing system with seat selection and PDF ticket generation.
- Implement rate‑limiting middleware for a Gin application.
- Create a social media clone backend with follow/unfollow and post feeds.
- Build a micro‑frontend architecture integrating Go APIs with a React UI.
- Develop a video streaming service with HLS support and concurrent Go routines.
- Implement payment processing integration with Stripe in a web app.
- Create a job board API with search, filters, and pagination features.
- Build a booking system for hotels with date availability and reservation endpoints.
- Develop a weather dashboard that pulls external API data and caches responses.
- Implement dark mode support and user preferences stored in cookies.
- Create a file‑sharing service with expiring links and download tracking.
- Build a serverless web app using AWS Lambda with API Gateway (Go runtime).
- Develop a web crawler and present scraped data via a Gin interface.
- Implement multi‑tenant architecture in a web API with dynamic routing.
CLI Tools
- Build a command‑line TODO list manager with persistent storage in BoltDB.
- Create a file‑search CLI tool similar to
grep
with colorized output. - Develop a CLI for interacting with the GitHub API to list issues and PRs.
- Implement a CLI password manager with encryption using Go’s
crypto
package. - Build a static site generator that converts Markdown to HTML.
- Create a CLI tool to batch‑resize images using Go’s
image
package. - Develop a Docker‑like container runtime CLI using Linux namespaces.
- Implement a CLI to manage AWS resources using the AWS SDK for Go.
- Build a network‑scanner tool similar to Nmap.
- Create a CLI tool to convert file formats (CSV ↔ JSON, XML ↔ JSON).
- Develop a CLI for sending HTTP requests like
curl
but with JSON support. - Implement a CLI task scheduler that executes commands at set intervals.
- Build a CLI utility to monitor system metrics (CPU, memory, disk).
- Create a CLI text editor with basic editing features.
- Develop a CLI for Bitcoin price tracking with real‑time updates.
- Implement a CLI to encrypt/decrypt files with AES‑GCM.
- Build a CLI git‑commit message generator integrating AI suggestions.
- Create a CLI for database migrations in PostgreSQL.
- Develop a CLI that interacts with the Slack API to send messages.
- Implement a CLI‑based chat client for IRC networks.
- Build a CLI to manage Kubernetes clusters via
client-go
. - Create a CLI for spawning and managing VMs using libvirt.
- Develop a CLI bookmark manager that syncs with a remote API.
- Implement a CLI log analyzer to parse and summarize logs.
- Build a CLI that generates code snippets from templates.
- Create a CLI for customizing and applying dotfiles.
- Develop a CLI to monitor website uptime and send alerts.
- Implement a CLI for bulk renaming and organizing files.
- Build a CLI currency converter pulling rates from an API.
- Create a CLI to visualize JSON data as a tree structure.
Networking & Microservices
- Build a gRPC service for user management using Protocol Buffers.
- Develop a microservice architecture with service discovery via Consul.
- Implement an API gateway in Go with role‑based routing and rate limiting.
- Create a load balancer that distributes HTTP traffic to backend servers.
- Build a message‑queue service using RabbitMQ client in Go.
- Develop a distributed task queue with NSQ.
- Implement a service‑mesh sidecar proxy in Go with Envoy integration.
- Create a peer‑to‑peer chat application using libp2p.
- Build a WebRTC signaling server in Go.
- Develop a TCP proxy server that logs and forwards data.
- Implement a UDP‑based chat app demonstrating unreliable protocols.
- Create a network latency measurement tool using ICMP ping.
- Build a custom DNS server with caching and forwarding.
- Develop a rate‑limited, IP‑based dynamic firewall in Go.
- Implement an MQTT broker for IoT messaging.
- Create a distributed key‑value store using the Raft consensus algorithm.
- Build an image‑processing microservice with gRPC streaming.
- Develop a push‑notification service using HTTP/2 and APNs.
- Implement a WebSocket load‑tester to simulate concurrent connections.
- Create a reverse proxy with dynamic configuration from etcd.
- Build a service‑mesh control plane in Go.
- Develop an SNMP monitoring tool for network devices.
- Implement a custom TCP handshake to explore low‑level networking.
- Create a multicast chat application for a local network.
- Build a RESTful service that interacts with a Kafka message broker.
- Develop a microservice using GraphQL federation.
- Implement a ZeroMQ‑based pub/sub messaging system.
- Create a health‑check service that monitors other microservices.
- Build circuit‑breaker middleware for HTTP clients.
- Develop a distributed tracing system integrating OpenTelemetry.
Database & ORM
- Build a simple ORM using Go’s
reflect
package. - Create a database‑migration tool supporting MySQL and PostgreSQL.
- Develop a Go wrapper for SQLite with connection pooling.
- Implement a CRUD CLI for PostgreSQL databases.
- Build a caching layer using Redis for a DB‑backed app.
- Create a search‑index service using Bleve.
- Develop a time‑series DB client for InfluxDB.
- Implement a backup/restore utility for MongoDB.
- Build a change‑data‑capture tool listening to MySQL binlogs.
- Create a Go app that exports DB metrics to Prometheus.
- Develop an audit‑logging system with DB triggers.
- Implement full‑text search in PostgreSQL via Go.
- Create a data‑migration pipeline between two SQL databases.
- Build a sharding proxy distributing queries across multiple DBs.
- Develop a reactive data‑streaming app using PostgreSQL LISTEN/NOTIFY.
- Implement an ORM plugin for GORM to handle soft deletes.
- Create a schema‑version dashboard for DB migrations.
- Build a connection‑pool manager with dynamic scaling.
- Develop a Go client for Neo4j graph databases.
- Implement data encryption with transparent decryption layer.
- Create a type‑safe query‑builder library in Go.
- Build an analytics dashboard reading from ClickHouse.
- Develop a data‑archival service exporting old records to CSV.
- Implement optimistic locking in a REST API using ETags.
- Create a Go wrapper for AWS DynamoDB with batch ops.
- Build a DB proxy that logs slow queries.
- Develop a replication tool syncing two MongoDB clusters.
- Implement real‑time DB sync using WebSockets.
- Create a Go SDK for Cassandra with consistency levels.
- Build a multi‑model DB client supporting SQL and NoSQL.
Concurrency & Parallelism
- Build a pipeline that processes files concurrently using channels.
- Develop a worker pool to handle tasks with rate limiting.
- Implement a concurrent web crawler using goroutines and channels.
- Create a parallel image‑processing pipeline with
sync.WaitGroup
. - Build a fan‑in/fan‑out pattern example reading from multiple sources.
- Develop a concurrent merge sort algorithm in Go.
- Implement an in‑memory key‑value store safe for concurrent access.
- Create a parallel ZIP file extractor using goroutines.
- Build a concurrent prime‑number finder with channel communication.
- Develop a multi‑threaded chat server handling clients concurrently.
- Implement a thread‑safe queue with mutexes and condition variables.
- Create a concurrent download manager for parallel file downloads.
- Build a rate‑limited concurrent HTTP client.
- Develop a concurrent map implementation with shard locking.
- Implement a fan‑out web‑request dispatcher.
- Create a bounded worker pool with backpressure.
- Build a concurrent log aggregator merging logs from multiple sources.
- Develop parallel matrix multiplication in Go.
- Implement a concurrent breadth‑first search on a graph.
- Create a parallel file‑encryption utility.
- Build a concurrent scheduler executing timed tasks.
- Develop a goroutine‑leak detector tool.
- Implement a mock network stress tester with concurrency.
- Create a concurrent data‑transformation pipeline.
- Build a concurrent genetic‑algorithm simulation.
- Develop a concurrent chatbot with multiple handler routines.
- Implement a parallel crawler respecting
robots.txt
. - Create a thread‑safe cache with TTL expiry.
- Build a concurrent event‑driven simulator.
- Develop a concurrent task orchestrator with dynamic scaling.
DevOps & Automation
- Build a CI/CD pipeline tool that triggers builds on Git hooks.
- Create an IaC CLI for provisioning servers via Terraform API.
- Develop a Kubernetes operator in Go to manage custom resources.
- Implement a Docker‑image vulnerability scanner.
- Build a server‑health checker that posts alerts to Slack.
- Create a backup automation tool for cloud storage buckets.
- Develop a log‑shipping agent sending logs to Elasticsearch.
- Implement a custom Prometheus exporter for system stats.
- Create a deployment tool with canary‑release support.
- Build a Go program that rotates logs and manages retention.
- Develop an automated SSL‑certificate renewal tool using Let’s Encrypt.
- Implement a chaos‑engineering tool to simulate failures.
- Create a container‑registry cleaner to remove old images.
- Build a resource‑usage dashboard using Go and D3.js.
- Develop a pipeline to automate DB migrations on deploy.
- Implement a GitOps agent syncing Git with Kubernetes.
- Create an auto‑scaling controller based on custom metrics.
- Build an incident‑management runbook executor for SRE.
- Develop a policy‑enforcer tool with OPA integration.
- Implement a secrets‑management CLI interacting with Vault.
- Create a monitoring dashboard via the Grafana HTTP API.
- Build a network‑configuration auditor for compliance.
- Develop a static code‑analysis tool for Go projects.
- Implement a concurrency‑test harness for microservices.
- Create a cost‑analysis tool for AWS usage.
- Build a configuration‑drift detector for servers.
- Develop a Kubernetes log aggregator with real‑time UI.
- Implement a multi‑cloud resource‑provisioning tool.
- Create a backup‑audit‑report generator.
- Build a deploy‑rollback utility with state tracking.
Data Processing & Analytics
- Build an ETL pipeline that reads CSV files and writes to PostgreSQL.
- Create a real‑time data‑processing app using Kafka consumer.
- Develop a data‑transformation tool with Go’s
encoding/json
and CSV. - Implement a clickstream‑analyzer parsing web log files.
- Build a sentiment‑analysis service integrating with a Python model via RPC.
- Develop a data‑deduplication utility for large datasets.
- Create a Go program that generates PDF data reports with charts.
- Implement a MapReduce framework for text processing in Go.
- Build a time‑series data aggregator for IoT sensor feeds.
- Develop a CSV‑to‑Parquet converter tool.
- Implement a Go client for Apache Spark’s REST API.
- Create a data‑quality checker validating JSON schemas.
- Build a real‑time fraud‑detection microservice.
- Develop a Go scraper that stores data in Elasticsearch.
- Implement a topic‑modeling tool using LDA in Go.
- Create a data‑anonymization utility for sensitive info.
- Build a financial‑data analysis toolkit pulling stock quotes.
- Develop a Go service for geospatial‑data indexing.
- Implement a recommendation engine using collaborative filtering.
- Create a query-optimizer demo for large datasets.
- Build a workflow engine for data pipelines.
- Develop a Go plugin for Apache Beam.
- Implement a data‑lineage tracker for ETL jobs.
- Create a data‑visualization web app with Go backend and Chart.js.
- Build an A/B‑testing analysis tool.
- Develop an OLAP cube generator in Go.
- Implement a streaming‑analytics dashboard with WebSockets.
- Create a pub/sub data pipeline using Google Pub/Sub.
- Build a log‑correlation tool for multi‑source logs.
- Develop a predictive‑maintenance service using a Go‑wrapped ML model.
Cloud & Serverless
- Build an AWS Lambda function in Go for image resizing.
- Create a GCP Cloud Function in Go to process Pub/Sub messages.
- Develop a CLI to deploy serverless apps to Azure Functions.
- Implement an autoscaler on Kubernetes using KEDA and Go.
- Create a serverless web app with Netlify Functions (Go).
- Build a Go API integrating with Firebase Firestore.
- Develop a FaaS orchestration tool for multi‑cloud environments.
- Implement a Go client for DigitalOcean Spaces.
- Create a static‑site deployer to S3 with CloudFront invalidation.
- Build a Go program that manages Kubernetes via
client-go
. - Develop a serverless image‑processing pipeline with AWS Lambda & S3.
- Implement a cost‑monitoring Lambda function that sends alerts.
- Create an event‑driven microservice using Azure Event Grid.
- Build a serverless Slack chatbot using AWS Lambda.
- Develop a Go app to deploy containers on AWS Fargate.
- Implement an edge function in Cloudflare Workers with Go/Wasm.
- Create a tool to migrate serverless functions between providers.
- Build a cloud‑resource tag auditor for cost optimization.
- Develop a serverless video transcoding service.
- Implement a Go program managing secrets in AWS Secrets Manager.
- Create a serverless GraphQL API with AWS AppSync.
- Build a Go‑based log processor in Google Cloud Dataflow.
- Develop a serverless notification service using SNS + Lambda.
- Implement a Go client for Alibaba Cloud OSS.
- Create a multi‑cloud DNS updater in Go.
- Build a serverless email service using AWS SES.
- Develop a Go tool for automated cert provisioning in cloud.
- Implement a serverless cron job scheduler using Step Functions.
- Create a Go plugin for Terraform to manage custom resources.
- Build a cloud‑cost optimization suggestion engine.
Security & Cryptography
- Implement a CLI to generate and manage SSH keys.
- Build a password‑hashing library using bcrypt and scrypt.
- Develop an OAuth2 server in Go with token storage.
- Create JWT‑authentication middleware for HTTP servers.
- Implement a TLS‑termination proxy for secure traffic.
- Build a certificate‑authority management tool.
- Develop a Go library for two‑factor authentication (TOTP).
- Create a code‑scanner tool to detect security vulnerabilities.
- Implement file encryption‑at‑rest with AES‑256‑GCM.
- Build a firewall‑configuration manager using
iptables
. - Develop a Kubernetes RBAC‑audit tool.
- Create a secure file‑sharing service with end‑to‑end encryption.
- Implement a password‑strength checker library.
- Build a Go client for Vault’s encryption API.
- Develop a phishing‑detector using regex and ML integration.
- Create a network packet sniffer and analyzer.
- Implement a secure chat app with the Signal protocol.
- Build a binary‑signing and verification tool.
- Develop a YARA‑rule scanner for malware detection.
- Create a Go app to monitor SSL/TLS cert expiry.
- Implement a Diffie‑Hellman key‑exchange demo.
- Build a Go library for homomorphic‑encryption basics.
- Develop a CVE‑tracker for Go dependencies.
- Create a passwordless login system with magic links.
- Implement a web‑app penetration‑test CLI.
- Build a backend for a browser extension that filters content.
- Develop a sandboxed code‑execution environment.
- Create a tool to encrypt HTTP cookies securely.
- Implement HMAC‑based request signing middleware.
- Build a secure file vault with multi‑user audit logs.
Systems Programming
- Build a simple OS kernel in Go using TinyGo and WebAssembly.
- Develop a virtual‑file‑system layer for custom file types.
- Implement a memory‑allocator in Go for educational purposes.
- Create a minimal hypervisor controller using Go.
- Build a device‑driver interface simulator.
- Develop a file‑system watcher using
fsnotify
. - Implement a simplified
curl
in Go using raw syscalls. - Create a process‑manager akin to Supervisord.
- Build a minimal shell in Go supporting scripting.
- Develop a Go library to parse ELF binaries.
- Implement a custom memory‑mapped file reader.
- Create a Go‑based debugger interface for processes.
- Build a system‑call tracer similar to
strace
. - Develop a resource‑limit (ulimit) manager.
- Implement Go interfaces to control Linux capabilities.
- Create a secure‑enclave simulator for confidential computing.
- Build a custom scheduler for goroutines at user level.
- Develop a Go library for interacting with
/proc
filesystem. - Implement a block‑device simulator.
- Create a power‑management daemon for servers.
- Build a performance‑profiler tool using
pprof
. - Develop a Go library for ACPI table parsing.
- Implement a network‑file‑system client.
- Create a crashed‑process analyzer for core dumps.
- Build a watchdog daemon that restarts failed services.
- Develop a Go tool to inspect cgroups resource usage.
- Implement a USB‑device enumeration utility.
- Create a Go wrapper over the Linux audit subsystem.
- Build an in‑memory database engine in Go.
- Develop a custom Go‑runtime feature plugin.
How to Come Up with Golang Project Ideas
- Identify a Need
- Look for everyday annoyances: slow file transfers, manual log analysis, etc.
- Explore Go Ecosystem
- Browse GitHub or pkg.go.dev to see popular libraries. What’s missing?
- Mix Interests
- Combine Go with hobbies: build a bot for your favorite game, or a data‑scraper for a sports site.
- Learn from Others
- Check out Go meetup talks or blogs. What fun side‑projects do people share?
Benefits of Doing Golang Projects
- Hands‑On Learning: You really understand Go’s syntax and tools.
- Confidence Boost: Finishing projects makes you proud and motivated.
- Networking: Share your code; get feedback from the Go community.
- Resume Power: Concrete projects speak louder than just “I know Go.”
Tips for Choosing the Best Golang Project
- Start Small: Pick a project you can finish in a few days or weeks.
- Match Your Level: Beginners should avoid giant distributed systems!
- Set Clear Goals: “Build a CLI to manage my to‑do list,” not “Write something in Go.”
- Use Version Control: Put every project on GitHub from day one.
- Document As You Go: Write README and comments—future you (and employers) will thank you!
Tools & Libraries to Know
- Gin (web framework)
- Cobra & Viper (CLI apps)
- GORM (ORM for databases)
- Testify (testing helpers)
- Go Modules (dependency management)
Step‑by‑Step: From Idea to Deployment
- Plan your features and tech stack.
- Initialize with
go mod init
. - Write code using small, testable functions.
- Test with Go’s built‑in testing (
go test
). - Document in README and comments.
- Build with
go build
. - Deploy to Heroku, DigitalOcean, or a VM.
Common Pitfalls & How to Avoid Them
- Ignoring Errors: Always check
err
after calls. - Blocking Goroutines: Use buffered channels or
select
. - Poor Module Management: Commit
go.mod
andgo.sum
early.
Next Steps: Sharing and Maintaining Your Project
- Open‑Source It: Add a license and push to GitHub.
- Write a Blog Post: Explain your learning journey.
- Iterate: Add features, fix bugs, and update docs.
Keep exploring and building—with every Golang project, you’ll grow into a stronger, more confident developer!